Detects and optionally removes a scan border from an image. A scan border is any border that was added by a scanning process. This subcode assumes that the border to be removed is dark, while the page is light. The border will be detected during REQ_INIT and removed during REQ_EXEC.
Occasionally, a border will not be detected. In those cases, the confidence of the detection will usually be low and the caller will usually skip the call to REQ_EXEC.
A number of parameters can be used to control the border detection process. While the detection process is tolerant of incorrect control values, setting these values correctly will provide more accurate results. In particular, setting a tight limit on the width and height of the page will produce more accurate results.
There are three options for removing a border: deskew, fill, and crop. Each can be used independently or in conjunction with the rest. At least one must be set to true.
Setting u.SC21.DeskewBorder to true will cause the output image to be deskewed, based on the angle of the detected border. In some cases, the content of a page will be skewed differently from the edge of the page. This subcode ignores the skew of the content of the page.
Setting u.SC21.ReplaceBorder to true will cause the border pixels to be replaced with new pixels, whose color matches PadColor.
Setting u.SC21.RemoveBorder to true will cause the border pixels to be removed from the image.
The images below demonstrate the operation of the subcode using various combinations of the above parameters.
→ (deskew) 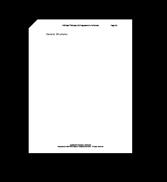
→ (remove) 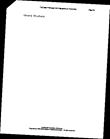
→ (replace) 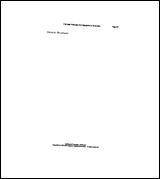
→ (deskew & replace) 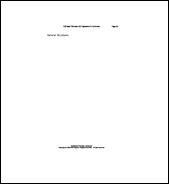
→ (deskew & remove) 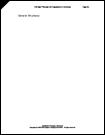
→ (replace and remove) 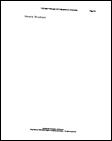
→ (deskew, remove, and replace) 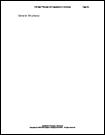
This subcode is unlike the other subcodes, in that it performs significant work during both REQ_INIT and REQ_EXEC. During REQ_INIT, it will read the image and examine it to find the border. At this time, it will also determine the size of the output image. During REQ_EXEC, it will again read the image and then crop and deskew the image to remove the border which was detected during REQ_INIT. All input values must be set before REQ_INIT. In some cases (such as u.SC21.DeskewImage), the operation will not be applied until REQ_EXEC, but the value is also used by REQ_INIT to determine the size of the output image.
The uncompressed image to be processed must reside in the Get Queue prior to both REQ_INIT and REQ_EXEC. Typically, the image will be fully contained in the Get Queue, but that is not a requirement. The output image will be placed into the Put Queue during REQ_EXEC.
The uncompressed image must be a minimum of 64x64 pixels or the error code ERR_BAD_MIN_SIZE will be returned during REQ_INIT.
Padding pixels present at the end of each line may be modified by this operation.
- Subcode is set to SF_SUBCODE_REMOVE_BORDER (21).
- DetectionQuality is set from 0 to 100, with 0 causing detection to run as fast as possible and 100 causing detection to be as careful and accurate as possible. 50 is a good default value.
- PadColor is set to the color to be used when pixels are added to the image. Pixels of this color will be added in several situations: If u.SC21.DeskewImage is true, some pixels may be added along the edges. If u.SC21.ReplaceBorder is true, some border pixels may be replaced with new pixels. If u.SC21.AmountToExpandPage is set to a value larger then the thickness of the border already present in the image, some pixels may be added to create a border of the desired thickness.
- u.SC21.BorderSpeckSize is set to the width or height, in pixels, of specks that reside within the border. Valid values range from 0 to 20. A good default value is 3.
- u.SC21.PageSpeckSize is set to the width or height, in pixels, of specks that reside within the border. Valid values range from 0 to 20. A good default value is 3.
- u.SC21.MinimumPageWidth is set to the minimum expected width, in pixels, of the page. Valid values range from 0 to 2,147,483,647. If the width of the page is unknown, this value should be set to 0. If no page can be found of the expected size, the detected page may lie outside the specified size. Typically, the confidence will be low in that case.
- u.SC21.MaximumPageWidth is set to the maximum expected width, in pixels, of the page. Valid values range from 0 to 2,147,483,647. If the width of the page is unknown, this value should be set to 2,147,483,647. If no page can be found of the expected size, the detected page may lie outside the specified size. Typically, the confidence will be low in that case.
- u.SC21.MinimumPageHeight is set to the minimum expected height, in pixels, of the page. Valid values range from 0 to 2,147,483,647. If the height of the page is unknown, this value should be set to 0. If no page can be found of the expected size, the detected page may lie outside the specified size. Typically, the confidence will be low in that case.
- u.SC21.MaximumPageHeight is set to the maximum expected height, in pixels, of the page. Valid values range from 0 to 2,147,483,647. If the height of the page is unknown, this value should be set to 2,147,483,647. If no page can be found of the expected size, the detected page may lie outside the specified size. Typically, the confidence will be low in that case.
- u.SC21.AmountToExpandPage is set to the number of pixels to expand each of the four page edges. If this value is set to a negative value, additional pixels will be removed from the image. This value should have a small positive value if some part of the border is desired in the output image. Valid values range from -1000 to 1000, with zero being a good default. In some situations, it is important that the output image have some small visual indication of the location of a border that was removed. For those situations, this value can be set to a small positive value, such as 10. In other situations, it is important that all sign of the border be removed. For those situations, this value can be set to a small negative value, such as -5. Note that this value must be set before REQ_INIT so the size of the output image can be determined.
- u.SC21.DeskewBorder is set to true to indicate that the image should be deskewed (based on the angle of the detected border.) Valid values are true and false.
- u.SC21.ReplaceBorder is set to true to indicate that border pixels (which remain in the output image) should be replaced with pixels that match PadColor. Valid values are true and false.
- u.SC21.RemoveBorder is set to true to indicate that border pixels should be removed from the output image. Since pages are rarely perfectly rectangular, some border pixels will usually remain after the border is removed.
- Stride is set to the image line width in bytes including padding at the end of the line width or to 0. If Stride is 0 and PF_NoDibPad is set in PicFlags, the operation will compute the stride using: [ (PixelWidth*BitCount)/8 ]. If Stride is 0 and PF_NoDibPad is not set in PicFlags, the operation will compute the stride using: [ (((PixelWidth*BitCount)+31)&(~31))/8 ].
In addition to placing an output image into the Put Queue, this operation stores some output information into the PIC_PARM structure in the following locations.
- Confidence is set, during REQ_INIT, from 0 to 100 with 0 indicating that the detected border is probably not correct and 100 indicating that the detected border is almost certainly correct. Values above 70 usually indicate accurate results and values below 50 frequently indicate incorrect results. Depending on the needs of the application, this value can be used to recognize and redirect problem images before too much time is invested in them.
- u.SC21.DetectedHorizontalAngle is set, during REQ_INIT, to the amount of rotation (in degrees counter-clockwise) needed to correct the skew of horizontal lines in the image. Generally, this will be identical to, or nearly identical to u.SC21.DetectedVerticalAngle. This value will always be in the range -20 to +20 inclusive.
- u.SC21.DetectedVerticalAngle is set, during REQ_EXEC, to the amount of rotation (in degrees counter-clockwise) needed to correct the skew of vertical lines in the image. Generally, this will be identical to, or nearly identical to u.SC21.DetectedHorizontalAngle. This value will always be in the range -20 to +20 inclusive.
- u.SC21.PageX is set, during REQ_INIT, to the X coordinate of the top left corner of the detected page. This value will usually be between 0 and the width of the input image, in pixels. In rare cases, this value may be negative, indicating that the page was clipped during scanning.
- u.SC21.PageY is set, during REQ_INIT, to the Y coordinate of the top left corner of the detected page. This value will usually be between 0 and the height of the input image, in pixels. In rare cases, this value may be negative, indicating that the page was clipped during scanning.
- u.SC21.PageWidth is set, during REQ_INIT, to the width of the detected page, in pixels. This value will be between 0 and the width of the input image.
- u.SC21.PageHeight is set, during REQ_INIT, to the height of the detected page, in pixels. This value will be between 0 and the height of the input image.
- OutputStride is set, during REQ_INIT and REQ_EXEC, to the image line width in bytes including padding at the end of the line.
- BiOut is set, during REQ_INIT and REQ_EXEC, to the attributes of the output image. It will first be set to a copy of the Head structure with the PIC_PARM structure. Then, the biWidth, biHeight, and biSizeImage parameters will be adjusted (if necessary) to the new values.